How to build your own JSON REST API with Angular & PHP. This tutorial just show the R (read) from the CRUD application. The Create, Update and Delete Requests are similar files. You can define your own POST request parameters to change the data. Thats just a simple table listing of the read api.
Frontend
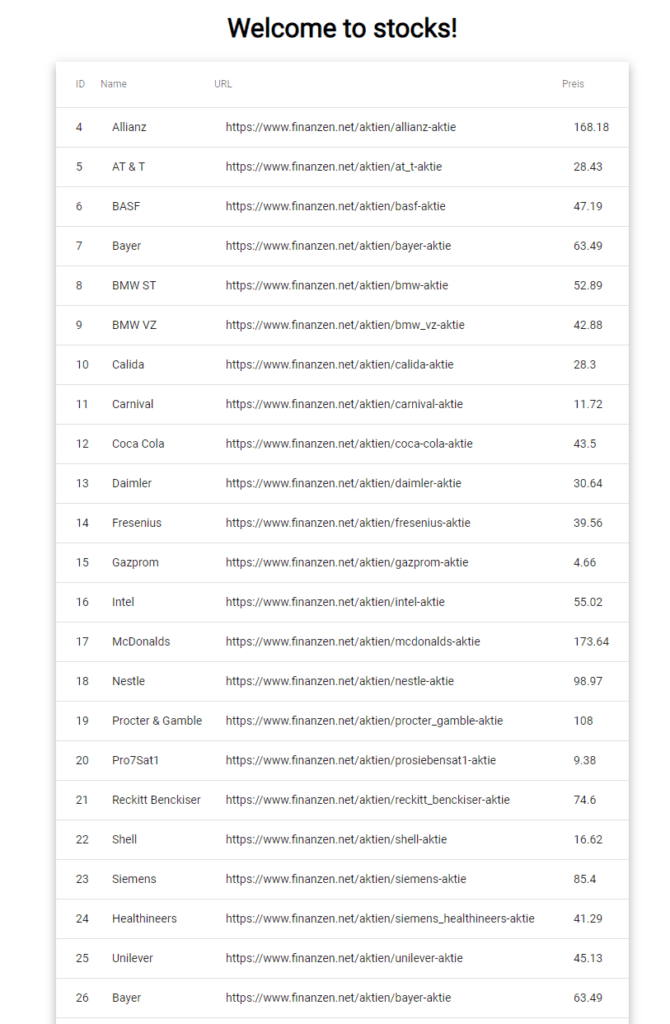
list-stocks.component.ts
import { Component, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-list-stocks', templateUrl: './list-stocks.component.html', styleUrls: ['./list-stocks.component.scss'] }) export class ListStocksComponent implements OnInit { displayedColumns: string[] = ['id', 'name', 'url', 'preis']; dataSource: string []; ngOnInit() { } private data:any = [] constructor(private http: HttpClient) { const url ='https://DEINEURL.net/api/read/' this.http.get(url).subscribe((res)=>{ this.data = res console.log(this.data) this.dataSource = this.data; }) } }
list-stocks.component.html
https://material.angular.io/components/table/overview
<table mat-table [dataSource]="dataSource" class="mat-elevation-z8"> <!--- Note that these columns can be defined in any order. The actual rendered columns are set as a property on the row definition" --> <!-- Position Column --> <ng-container matColumnDef="id"> <th mat-header-cell *matHeaderCellDef> ID </th> <td mat-cell *matCellDef="let element"> {{element.id}} </td> </ng-container> <!-- Name Column --> <ng-container matColumnDef="name"> <th mat-header-cell *matHeaderCellDef> Name </th> <td mat-cell *matCellDef="let element"> {{element.name}} </td> </ng-container> <!-- Weight Column --> <ng-container matColumnDef="url"> <th mat-header-cell *matHeaderCellDef> URL </th> <td mat-cell *matCellDef="let element"> {{element.url}} </td> </ng-container> <!-- Symbol Column --> <ng-container matColumnDef="preis"> <th mat-header-cell *matHeaderCellDef> Preis </th> <td mat-cell *matCellDef="let element"> {{element.preis}} </td> </ng-container> <tr mat-header-row *matHeaderRowDef="displayedColumns"></tr> <tr mat-row *matRowDef="let row; columns: displayedColumns;"></tr> </table>
list-stocks.component.scss
table.mat-table { margin: auto; } td.mat-cell { padding: 1em; }
Backend
Access Control Header wegen CORS
Das letzte Komma muss gelöscht werden, sonst ist es kein valides JSON.
<?php header('Access-Control-Allow-Origin: *'); include("../dbconnect.php"); $sql = "SELECT id, name, url, preis from aktien"; $result = $mysqli->query($sql); $json = "["; if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { $json .= json_encode($row).","; } } $json = substr($json, 0, -1); $json .= "]"; echo $json; ?>
JSON Parser
https://jsonformatter.org/json-parser
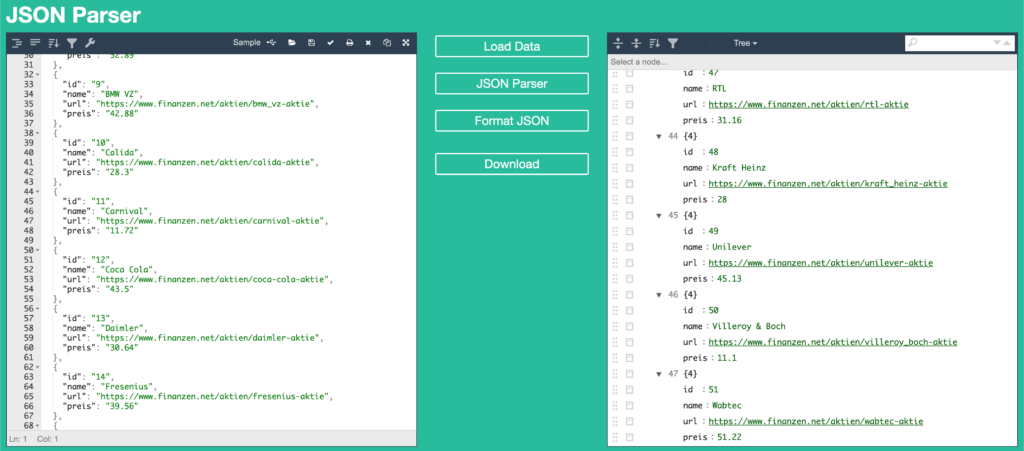
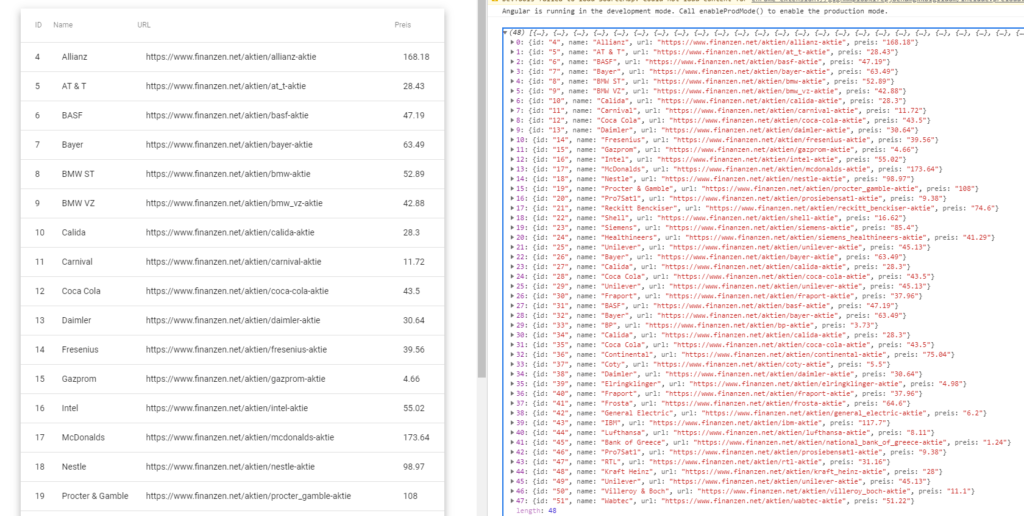